1. Understanding Common Errors
- Sujatha R
- Oct 24, 2023
- 1 min read
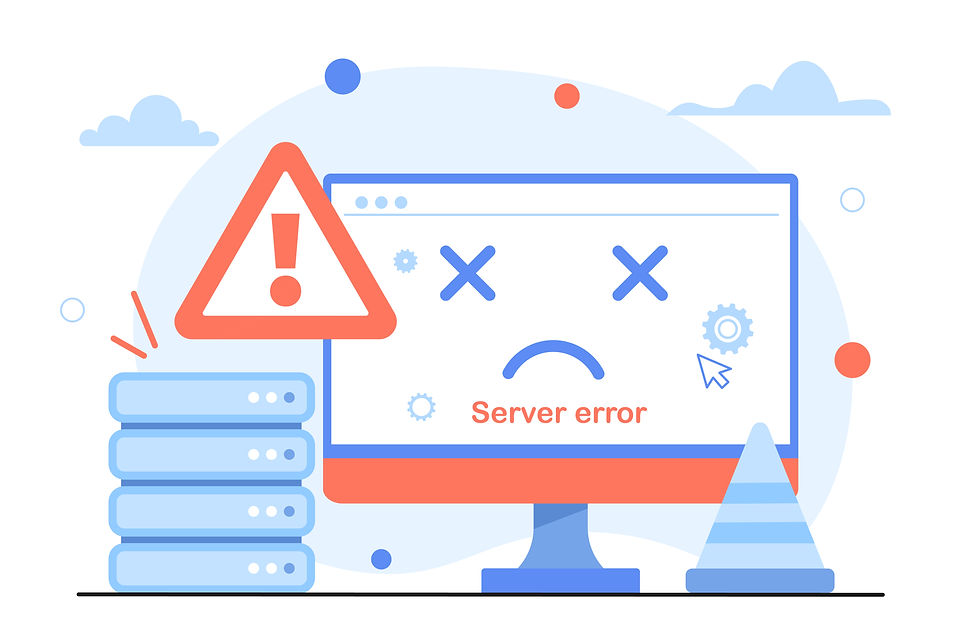
1.1. Network Connectivity Issues
One of the most common issues developers face is network connectivity. This can occur when a user's device is not connected to the internet or there are issues with their network configuration.
code
try {
const accounts = await ethereum.request({ method: 'eth_requestAccounts' });
} catch (error) {
if (error.code === 4100) {
// User rejected the request
console.log('Please connect to a network');
} else {
console.error(error);
}
}
1.2. User Denies Access
Users have the option to deny access to their accounts or specific operations. It's important to handle this scenario gracefully.
code
try {
const accounts = await ethereum.request({ method: 'eth_requestAccounts' });
} catch (error) {
if (error.code === 4001) {
// User rejected the request
console.log('User denied account access');
} else {
console.error(error);
}
}
1.3. Incorrect Network Selection
Users may not always be connected to the desired Ethereum network. Handling this situation involves verifying network ID and providing clear instructions for the user to switch networks.
code
const requiredNetwork = 1; // Mainnet
if (ethereum.networkVersion !== requiredNetwork.toString()) {
console.log('Please switch to the Mainnet network');
}
Comentários